이 프로젝트의 개발 환경
- 개발 언어 및 개발 환경
- OpenJDK 17
- 기타 환경
- macOS Sonoma 14.1.1
- IntelliJ IDEA 2020.3 Ultimate Edition
예제에 앞서 이 블로그의 문서: Chapter 02A. 스프링 프로젝트 시작하기를 선행합니다.
Greeter 클래스 생성
다음 경로 src/main/java에서 새로운 패키지 chap02를 생성합니다. 그리고 패키지 안에 Greeter.java 클래스를 생성합니다.
package chap02;
public class Greeter
{
private String format;
public String greet(String guest)
{
return String.format(format, guest);
}
public void setFormat(String format)
{
this.format = format;
}
}
예제의 Greeter 객체를 생성하고 관련 메소드를 호출하여 문자열 포멧을 지정하고 메시지를 생성합니다.
예를 들어, 예제를 따라가면 다음과 같이 Greeter 클래스를 사용하게 됩니다.
Greeter greeter = new Greeter();
greeter.setFormat("%s, 안녕하세요!");
// msg에는 "스프링, 안녕하세요!"가 저장됩니다.
String msg = greeter.greet("스프링");
AppContext 클래스 생성
chap02 패키지에서 AppContext.java 클래스를 생성합니다.
package chap02;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AppContext
{
@Bean
public Greeter greeter()
{
Greeter greeter = new Greeter();
greeter.setFormat("%s, 안녕하세요!");
return greeter;
}
}
코드 | 비고 | |
AppContext 클래스를 |
||
Spring에서 Bean 객체를 생성하는 메소드는 |
AppContext 클래스를 사용해 Bean 객체 생성하기
chap02 패키지에서 Main.java 클래스를 생성합니다.
package chap02;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Main
{
public static void main(String[] args)
{
AnnotationConfigApplicationContext ctx
= new AnnotationConfigApplicationContext(AppContext.class);
Greeter greeter = ctx.getBean("greeter", Greeter.class);
String msg = greeter.greet("스프링");
System.out.println(msg);
ctx.close();
}
}
코드 | 비고 | |
인자로 전달된 AppContext의 그리고 설정 정보를 통해 Bean 객체를 생성하고 초기화하고 관리합니다. |
||
Main 클래스를 우클릭 | Run 'Main.main()' 하여 앱을 실행하고 출력 결과를 확인합니다.
스프링, 안녕하세요!
싱글톤(Singleton) 객체
예제의 Bean 객체를 생성하는 코드 Main.java를 다음과 같이 수정합니다.
package chap02;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Main
{
public static void main(String[] args)
{
AnnotationConfigApplicationContext ctx
= new AnnotationConfigApplicationContext(AppContext.class);
Greeter greeter1 = ctx.getBean("greeter", Greeter.class);
Greeter greeter2 = ctx.getBean("greeter", Greeter.class);
System.out.println(greeter1 == greeter2);
ctx.close();
}
}
코드 | 비고 | |
두 개의 Bean 객체를 생성하고 비교합니다. |
Main 클래스를 우클릭 | Run 'Main.main()' 하여 앱을 실행하고 출력 결과를 확인합니다.
true
디폴트로 Bean 객체는 싱글톤(Singleton) 객체입니다.
별도 설정을 하지 않았을 경우 Spring은 @Bean 어노테이션에 대해서 한 개의 Bean 객체를 생성합니다.
정리 및 복습
- 예제에서 가장 중요한 코드는
AnnotationConfigAppliccationContext클래스입니다. - Spring의 핵심은 객체를 생성하고 초기화 하는 과정입니다. 이 과정은
ApplicationContext인터페이스에서 정의됩니다. AnnotationConfigAppliccationContext는ApplicationContext인터페이스를 구현하며 자바 클래스를 통해 @Bean 설정 정보를 읽습니다.- 그리고 읽어온 정보를 통해
Bean 객체를 생성 및 초기화합니다. - 별도 설정을 하지 않았을 경우 Spring은
@Bean 어노테이션에 대해서 한 개의 Bean 객체를 생성합니다.
다음은 AnnotationConfigAppliccationContext 클래스 계층도의 일부입니다.
계층도의 최상단에는 BeanFactory 인터페이스 위치합니다.
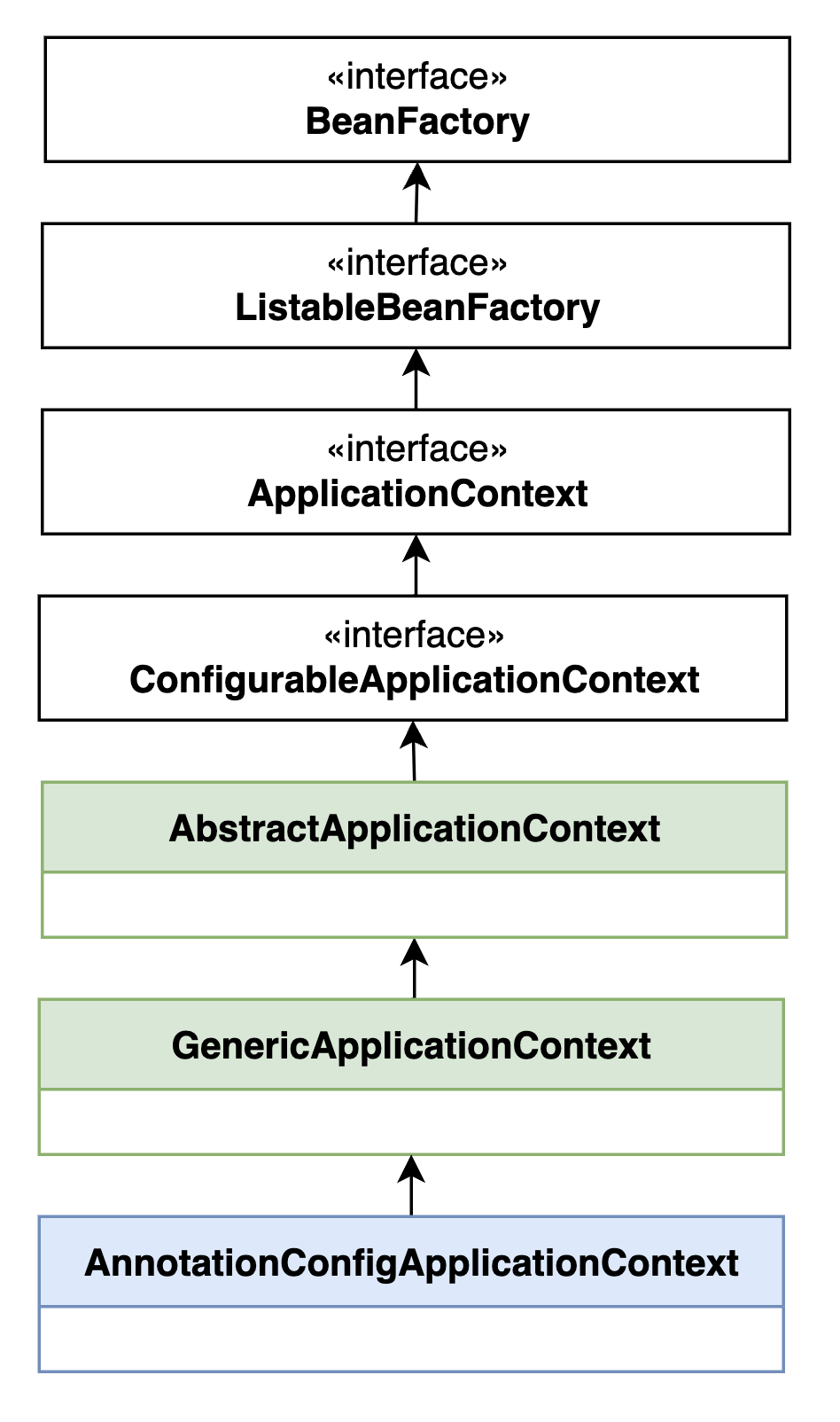
최하단 노드에 해당하는 AnnotationConfigAppliccationContext는 BeanFactory를 구현하거나 상속하는 인터페이스 및 클래스의 기능을 정의합니다.
동일한 레벨에서 유사한 기능을 하는 다른 Context도 존재합니다(e.g. Groovy 코드를 사용해 설정 정보를 가져오는 GenericGroobyApplicationContext 처럼).
ApplicationContext 또는 BeanFactory는 Bean 객체의 생성, 초기화, 보관, 제거 등을 관리하므로 Spring 컨테이너(Container)라고 부릅니다.
'Java > Spring' 카테고리의 다른 글
Spring Boot에서 실행되는 Tomcat 서버 포트 변경 방법 (0) | 2023.11.22 |
---|---|
Spring.io 따라하기: RESTful 웹 서비스에 요청하기 (0) | 2023.11.22 |
Spring.io 따라하기: RESTful 웹 서비스 구현하기 (0) | 2023.11.20 |
Spring 5 입문: Chapter 02A. 스프링 프로젝트 시작하기 (0) | 2023.11.17 |
"Could not resolve org.springframework.boot:spring-boot-gradle-plugin:3.x.x" 스프링 프레임워크 플러그인 오류 (0) | 2023.11.07 |